Unlocking Multi-Threading in JavaScript with Web Workers
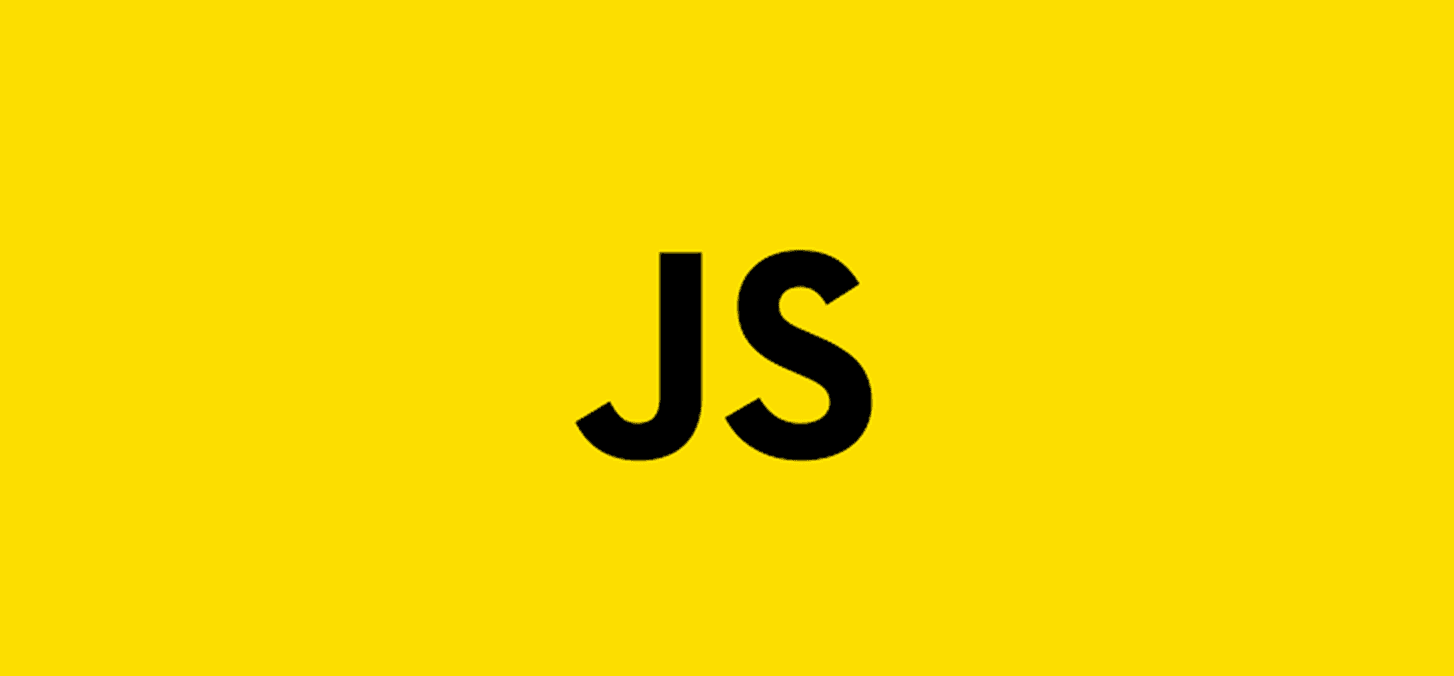
Since the beginning of my journey in the realm of front-end development, I always thought that JavaScript was strictly single-threaded. The idea of developing multi-threaded applications seemed out of reach, mainly because I didn't need it for the small calculations I was doing and thought more complex code would be too costly. I guess it wasn't very popular back then, and many developers might have faced the same limitations.
I want to talk about web API services like service worker and web worker and useful libraries to enhance performance on web apps. You can use Service Worker to create PWA apps that will install your JavaScript web app in the software or create a proxy to handle web app offline behavior. On the other hand, you can use web workers to export your calculation from the main thread this means that you can calculate and do your business without blocking the main thread.
However, as web applications grew more sophisticated, the need for better performance and responsiveness became critical. This is where Web Workers come into play, allowing us to perform complex computations without blocking the main thread. In this article, I will explore Web API services like Service Worker and Web Worker and discuss useful libraries to enhance web app performance. So, let's dig into the code and get our hands dirty.
Understanding Web Workers
Web Workers provide a way to run scripts in background threads. They are perfect for handling heavy computations, allowing the main thread to remain responsive. This means you can perform tasks like data processing, image manipulation, or other intensive tasks without causing the UI to freeze.
Here's a simple example of how to create a Web Worker:
// worker.js
onmessage = function(e) {
let result = e.data * 2; // Example computation
postMessage(result);
};
// main.js
const worker = new Worker('worker.js');
worker.onmessage = function(e) {
console.log('Result from worker: ', e.data);
};
worker.postMessage(10); // Sending data to the worker
In this example, we create a worker script (worker.js) that listens for messages, processes data, and sends the result back to the main script (main.js).
Enhancing Performance with Service Workers
Service Workers are a type of Web Worker that acts as a proxy between your web app and the network. They enable features like offline support, background sync, and push notifications, making them essential for Progressive Web Apps (PWAs).
Here's a basic example of registering a Service Worker:
// main.js
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/service-worker.js')
.then(function(registration) {
console.log('Service Worker registered with scope: ', registration.scope);
}).catch(function(error) {
console.log('Service Worker registration failed: ', error);
});
}
And a simple Service Worker script:
// service-worker.js
self.addEventListener('install', function(event) {
console.log('Service Worker installing.');
});
self.addEventListener('fetch', function(event) {
console.log('Fetching:', event.request.url);
});
This Service Worker script listens for the install event and logs network requests. You can extend this to cache assets and handle offline behavior, significantly improving your app's performance and user experience.
JavaScript Event Loop with web workers:
Deeper Details about web workers
- Thread Creation: the browser spawns a separate thread. This is a system-level thread distinct from the main JavaScript execution thread. It has its execution context, meaning it does not share the same global variables or scope as the main thread.
- Isolation: Each Web Worker operates in isolation from the main thread and other workers. They communicate with the main thread via a messaging system using the postMessage method and can handle messages through the onmessage event handler. This isolation ensures that the workers cannot directly access the DOM or other objects in the main execution context, thus providing a safe environment in which to perform concurrent tasks.
- Concurrency vs. Parallelism: While JavaScript in the main thread runs on a single-threaded event loop, Web Workers enable true concurrency by running on separate threads. However, whether these threads run in parallel depends on the underlying system's hardware capabilities, such as the number of CPU cores.
Useful Libraries and Tools
Several libraries and tools can help you leverage Web Workers and Service Workers more effectively:
- Comlink: Simplifies communication with Web Workers by providing a concise API.
- Workbox: A powerful library for managing caching strategies and other Service Worker tasks.
- TensorFlow.js: For machine learning tasks in Web Workers.
- Partytown: Helps offload third-party scripts to Web Workers, improving main thread performance.
Conclusion
JavaScript multi-threading is no longer a myth. You can build highly performant and responsive web applications with Web Workers and Service Workers. By offloading heavy computations to background threads and enhancing offline capabilities, you can significantly improve the user experience of your web apps. Embrace these tools and take your front-end development skills to the next level.
Happy coding!